Week 2 - Section 0 - Repetition is the mother of knowledge
Site: | ΕΛ/ΛΑΚ Moodle |
Course: | Python Lab - May 2017 |
Book: | Week 2 - Section 0 - Repetition is the mother of knowledge |
Printed by: | Guest user |
Date: | Monday, 17 February 2025, 5:02 PM |
Description
Concepts of week one repeated.
Chapter 0.1 - 2D Printing in Python
During last week we learned some new concepts that are used widely in Python.Let's remember them starting with Print command.
As we learned, Print is an output command which sends its results to the screen.
print("Hello World") sends the message Hello World to the screen.
Anything inside the parentheses will be printed on screen, if the syntax is right.
We can print plain text as the previous example (using always the quotes characters "" or ''),
simple numbers,
>>> print(42)
42
>>>
the results of algebric operations inside parentheses (using the standard algebric operations' priority),
>>> print(35*6+7**2)
259
>>>
or combinations of the above examples:
>>> print("The second power of 4 equals to",4**2)
The second power of 4 equals to 16
>>>
We can also declare that the separator between numbers will be a character instead of the space character:
>>> print(192,168,178,42,sep=".")
192.168.178.42
>>>
If we need to print something moving to a new line we use the escape code \n:
>>> print('First line.\nSecond line.')
First line.
Second line.
>>>
If we need to print a reserved character we use the character \ and after that the reserved character to be printed:
>>> print("The file is stored in C:\\new folder")
The file is stored in C:\new folder
>>>
Chapter 0.2 - No comments or more comments
So, what if you have a complicated coding idea that needs hundreds of coding lines to write and lots of hours to describe them to a friend?Taking notes onto the program is a good approach. Python gives this capability by using special character # and three single quotes '''
Examples:
# Everything in this line will not be executed
print("Hello")#Anything from this point will be ignored until changing line
'''
Everything between three single quotes will not be executed
'''
Chapter 0.3 - Assign a value (not a mission) to a variable
Variables are specific locations in computer's memory. We need to use them because we live in a rapid changing environment and we need to remember past values of anything we want to describe. Time, temperature, light are some of these changing concepts.Variables in Python are named by us and a smart tactic is to name a variable according to what they represent.
So light could be the name of the variable that we use to measure the light intensity of a room.
Be careful that names are case sensitive, so Light is a different variable than light.
We can not use reserved characters, space connected words or numbered started words as variable names.
Every variable belongs to a type, so we have string(text) variables, integer variables, floating point variables and others.
Python variables do not need explicit declaration to reserve memory space. The declaration happens automatically when you assign a value to a variable. The equal sign (=) is used to assign values to variables.
The operand to the left of the = operator is the name of the variable and the operand to the right of the = operator is the value stored in the variable.
counter = 100 # An integer assignment miles = 1000.543 # A floating point name = "John" # A string
type() function returns the type of a variable. For example:
>>> type(counter)
<class 'int'>
>>>
Python allows you to assign a single value to several variables simultaneously.
a = b = c = 1
Chapter 0.4 - Read my keyboard
We learnt earlier how we can assign a value directly to a variable. What if we need to assign a value to a variable, reading it from the keyboard? Input() function does the trick.We can include text inside the parentheses, so that the system guides the user giving instructions.
For example:
>>> input("What's your name? ")
What's your name? John
'John'
>>>
As you notice Python asks for the name, user gives it and finally Python prints it on screen using single quotes.
If you need to input the name directly into a variable the syntax should be changed as following:
>>> myname=input("What's your name? ")
What's your name? John
>>>
Of course we can input a numbered value into a variable, but be careful!
Input() assigns always strings(text) into a variable.
So, using age=input("What is your age? ") and assuming the user responds with 30, then the age variable is a string variable which equals to "30".
We can not make arithmetic operations with this variable. If we need to use it as arithmetic operand, we have to convert it firstly to an integer variable using:
>>> age=int(age)
But there is a shortcut in all this. We can use just one command to input and convert our input at once:
>>> age=int(input("What is your age? "))
What is your age? 30
>>>
"Hit two birds with one stone!"
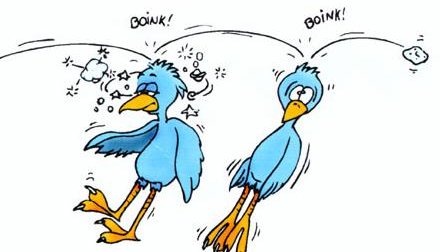