Book
Week 2 - Section 0 - Repetition is the mother of knowledge
Week 2 - Section 0 - Repetition is the mother of knowledge
Concepts of week one repeated.
Chapter 0.4 - Read my keyboard
We learnt earlier how we can assign a value directly to a variable. What if we need to assign a value to a variable, reading it from the keyboard? Input() function does the trick.
We can include text inside the parentheses, so that the system guides the user giving instructions.
For example:
>>> input("What's your name? ")
What's your name? John
'John'
>>>
As you notice Python asks for the name, user gives it and finally Python prints it on screen using single quotes.
If you need to input the name directly into a variable the syntax should be changed as following:
>>> myname=input("What's your name? ")
What's your name? John
>>>
Of course we can input a numbered value into a variable, but be careful!
Input() assigns always strings(text) into a variable.
So, using age=input("What is your age? ") and assuming the user responds with 30, then the age variable is a string variable which equals to "30".
We can not make arithmetic operations with this variable. If we need to use it as arithmetic operand, we have to convert it firstly to an integer variable using:
>>> age=int(age)
But there is a shortcut in all this. We can use just one command to input and convert our input at once:
>>> age=int(input("What is your age? "))
What is your age? 30
>>>
"Hit two birds with one stone!"
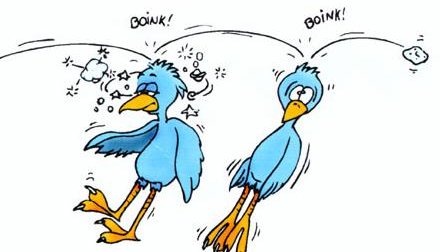
We can include text inside the parentheses, so that the system guides the user giving instructions.
For example:
>>> input("What's your name? ")
What's your name? John
'John'
>>>
As you notice Python asks for the name, user gives it and finally Python prints it on screen using single quotes.
If you need to input the name directly into a variable the syntax should be changed as following:
>>> myname=input("What's your name? ")
What's your name? John
>>>
Of course we can input a numbered value into a variable, but be careful!
Input() assigns always strings(text) into a variable.
So, using age=input("What is your age? ") and assuming the user responds with 30, then the age variable is a string variable which equals to "30".
We can not make arithmetic operations with this variable. If we need to use it as arithmetic operand, we have to convert it firstly to an integer variable using:
>>> age=int(age)
But there is a shortcut in all this. We can use just one command to input and convert our input at once:
>>> age=int(input("What is your age? "))
What is your age? 30
>>>
"Hit two birds with one stone!"
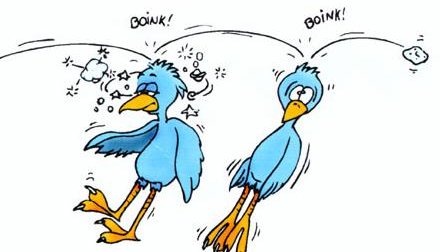